Crafting Effective Pull Requests: A Guide to Collaborative Development
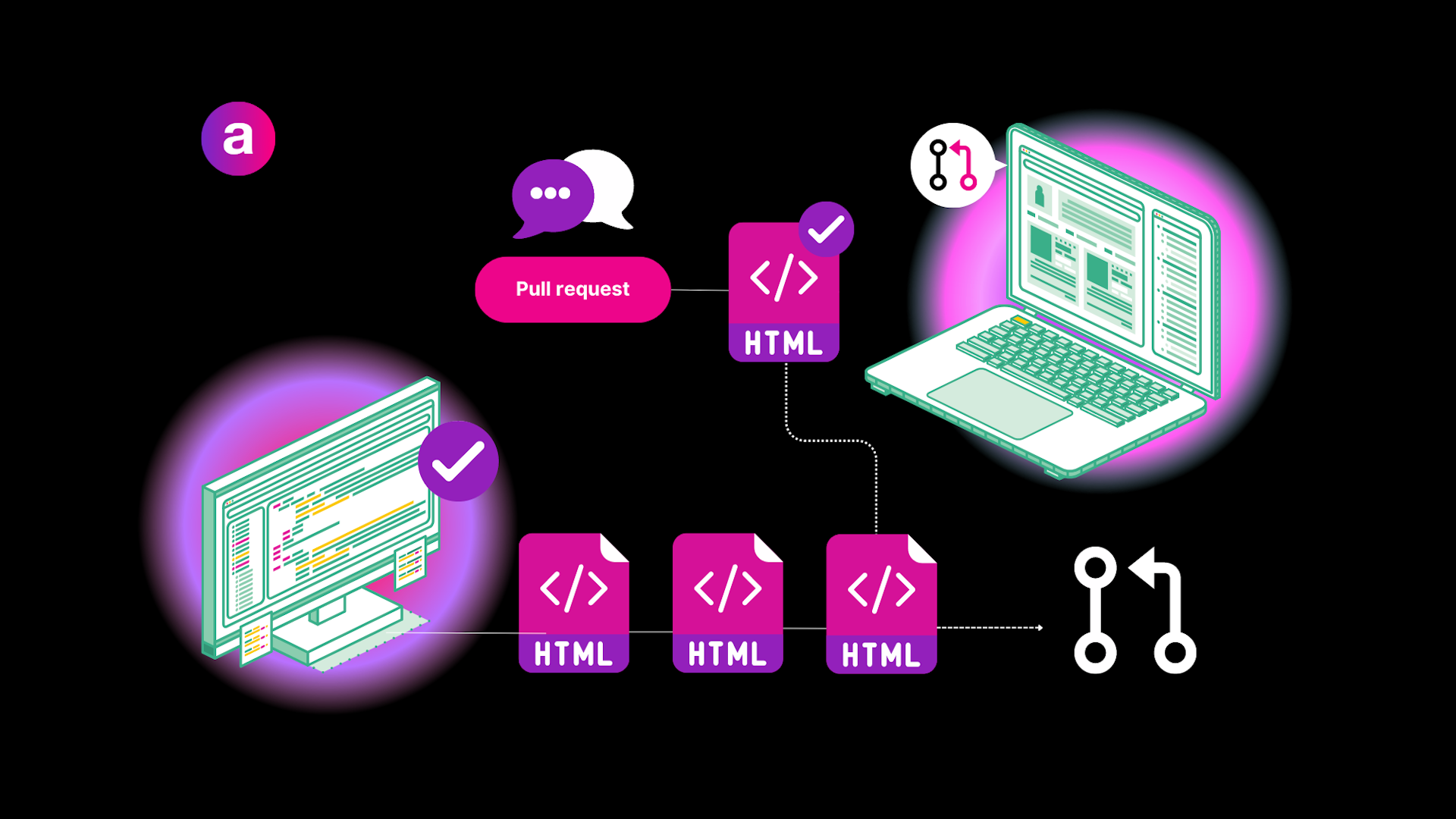
Developers often collaborate on projects, and one of the most essential tools for collaboration is creating pull requests (PRs). PRs are a quintessential part of asynchronous communication in software development. By honing your skills in making effective PR's, you will not only contribute to the team's success, but also accelerate your own growth as a developer. This guide will discuss six essential practices that help in making effective PRs to make you become a great software engineer.
1. Keep PRs Small and Focused
It's important to keep pull requests small and focused on a specific change or feature. This makes it easier for reviewers to understand your changes and provide valuable feedback. When a PR is too large, it becomes challenging to ensure that all changes are thoroughly reviewed. By keeping your pull requests small, you increase the likelihood of receiving detailed feedback and expedite the process of getting your changes merged.
Let's say you are working on a feature that involves adding a new page to a web application. Instead of creating one large PR that includes all the changes related to the new page, you can break it down into smaller PR's that build off of each other. Here's a visual representation of how this could work:
Create PR #1 that adds the basic HTML and CSS for the new page. The base branch would be main/master.
Create PR #2 that adds JavaScript functionality and interactivity to the new page. The base branch would the the branch for PR #1
Create PR #3 that adds server-side functionality and database integration to the new page. The base branch would be the branch for PR #2.
Each PR builds off of the previous one, so the changes are incremental and easier to review. This approach also allows you to get feedback and make changes more quickly and efficiently.
2. Use Feature Flags
Feature flags are a powerful way to control the release of new features or changes in your application. They allow you to enable or disable specific functionality without modifying the code. By using feature flags in your pull request, you give your team the flexibility to test and release your changes gradually, reducing the risk of introducing bugs or issues. This is because the feature doesn’t have to be fully completed before merging.
if (featureFlagEnabled('newFeature')) {
// Code for the new feature
} else {
// Code for the old feature
}
function featureFlagEnabled(flagName) {
// Call an API to check if the feature flag is enabled
// Return true or false based on the response
}
Copied to clipboard!
3. Include a Loom Video Demonstrating Functionality
A picture is worth a thousand words, and a video is worth even more. Using a tool like Loom, you can quickly create a video demonstrating the functionality of your changes. Including a video in the PR description helps reviewers understand the impact of your changes, making it easier for them to provide meaningful feedback. In the description of the PR, make sure to also link the associated issue, provide information about any remaining work to be done, and any what kind of cases you tested including any edge cases.
4. Use Git Rebase Interactive for a Clean Git History
Maintaining a clean git history is essential for tracking changes and finding the source of bugs or issues. Using
git rebase interactive
Copied to clipboard!
You can re-organize, squash, or edit your commits, making the history easy to read and understand. A clean git history makes it easier for your team to review your changes and understand the evolution of your codebase.
git rebase -i master
Copied to clipboard!
This will open up an interactive editor that shows a list of all the commits on your branch, starting with the oldest one.
In the editor, you can change the action for each commit to one of several options:
pick
: keep the commit as issquash
ors
: combine the commit with the previous onereword
orr
: change the commit messagedrop
ord
: remove the commit entirely
For example, let's say you want to squash the last two commits together, reword the commit message for the third commit, and drop the fourth commit entirely. You would modify the file to look like this:
pick 1234567 First commit
squash abcdefg Second commit
reword hijklmn Third commit
drop opqrst Fourth commit
Copied to clipboard!
Here's how to save and exit the Vim editor when using git rebase -i
:
After running
git rebase -i
the interactive rebase file will open in Vim.Make your changes to the file as needed. Once you're done, press the
esc
key to exit insert mode and return to command mode.To save your changes and exit Vim, type
:wq
and press enter. This command will write the changes to the file and quit Vim.If you only want to save your changes without quitting Vim, you can use the
:w
command instead.If you made a mistake and want to discard your changes and exit Vim, type
:q!
and press enter. This command will discard your changes and force quit Vim.Note that if you've made changes that you want to keep, you should use
:wq
instead of:q!
.Once you've saved or discarded your changes and exited Vim, the interactive rebase will continue running.
5. Leverage Comments Strategically
Comments can be added in two places: in the code itself, or in the pull request. It's important to use comments strategically to help others understand your changes.
When adding comments in the code, focus on explaining complex logic or decisions that may not be immediately apparent. These comments should provide additional information that is not obvious from just reading the code. Make sure the comments are clear and concise, and avoid adding comments that simply repeat what the code is doing.
In the pull request, comments can be used to ask questions or provide explanations about your changes. This can be helpful in providing context for the reviewer and helping them understand the purpose and impact of your changes. Avoid duplicating comments that are already in the code, and focus on providing high-level explanations of your changes.
Remember, comments should add value and help others understand your code. Use them effectively to make your code more readable and easier to understand.
Wrapping up
In conclusion, creating an effective pull request is an essential skill for any developer working in a collaborative environment. By keeping PRs small, referencing related PRs, using feature flags, including demonstration videos, maintaining a clean git history, and leveraging comments strategically, you can create pull requests that are easy to review and facilitate efficient collaboration among your team members.